JavaScript Execution Context
In JavaScript, everything happens within an execution context. JavaScript is a synchronous, single-threaded language, meaning it executes code one line at a time in a sequential manner.
Components of the Execution Context
JavaScript’s execution context consists of two main components:
Memory Component
- Stores variables, objects, and functions as key-value pairs.
Code Component
- Executes code line by line.
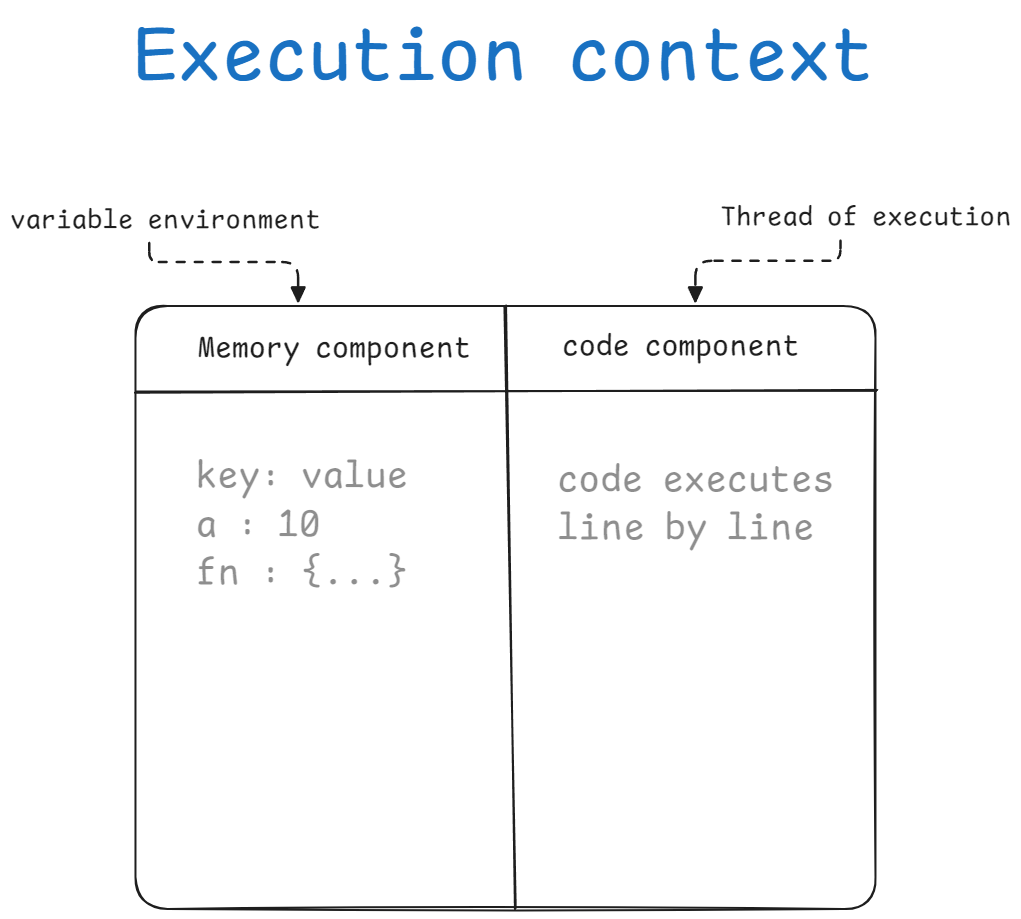
What Happens When You Run a JavaScript Program?
When you run a JavaScript program, an execution context is created. The execution context handles both the memory setup and the code execution.
How JavaScript Code is Executed
Consider the following JavaScript code:
var n = 2;
function square(num) {
var ans = num * num;
return ans;
}
var square2 = square(n);
var square4 = square(4);
Global Execution Context
When this code is executed, a global execution context is created with two phases:
1. Memory Creation Phase
- During this phase, memory is allocated for all variables and functions.
- Variables like
n
,square2
, andsquare4
are initially set toundefined
. - The function
square
is stored as a whole function.
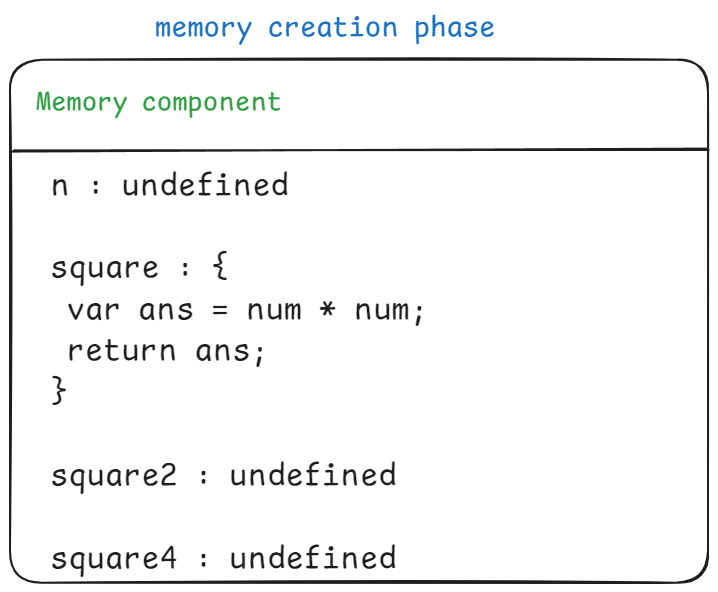
Code Execution Phase
After the memory is set up, the code is executed line by line:
- Line 1:
n = 2
- Line 8: A new execution context is created for the function
square(2)
:- Memory is assigned to the parameters
num
andans
. - Code execution calculates
ans = 4
. - The result
4
is returned, and the execution context is destroyed.
- Memory is assigned to the parameters
- Line 9: Another execution context is created for
square(4)
:- Memory is assigned to
num
andans
again. - Code execution calculates
ans = 16
. - The result
16
is returned, and the execution context is destroyed.
- Memory is assigned to
How Does JavaScript Manage Multiple Execution Contexts?
When there are nested function calls, JavaScript manages execution contexts through a call stack. This stack keeps track of the order of execution contexts, ensuring the correct context is called after the current one is completed.
For the code above, the call stack would look like this during execution:
